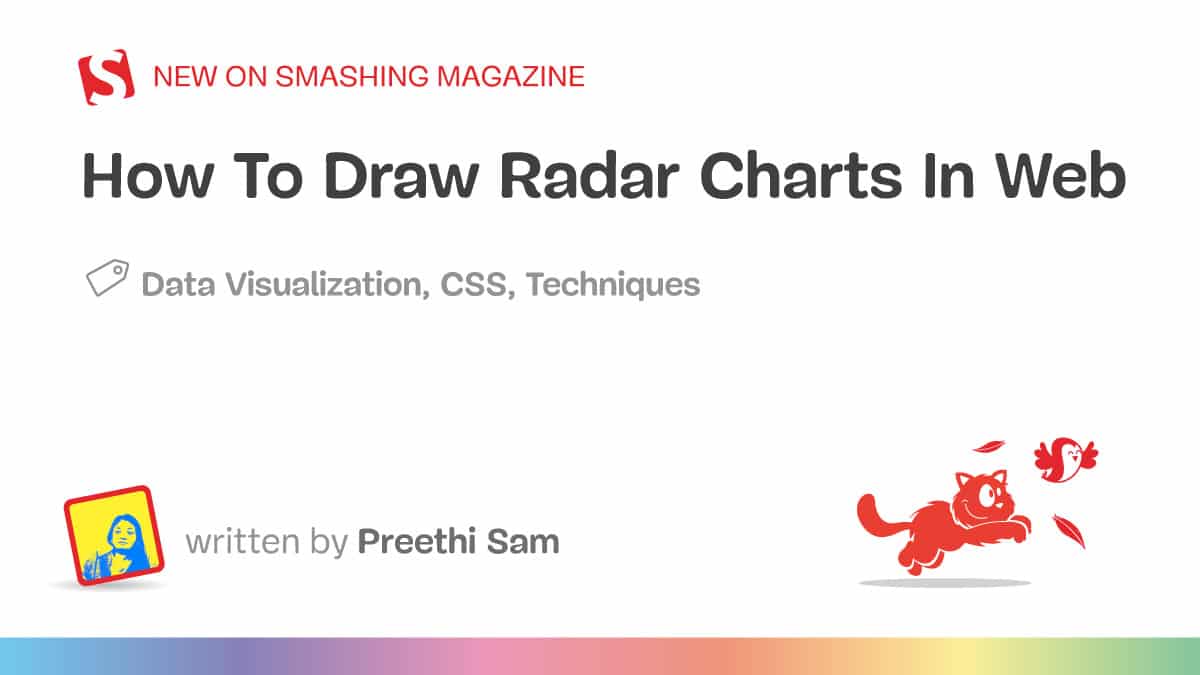
I set to work with a brand new sort of chart for knowledge visualization known as a radar chart when a mission requested for it. It was new to me, however the thought is that there’s a round, two-dimensional circle with plots going across the chart. Somewhat than easy X and Y axes, every plot on a radar chart is its personal axis, marking a spot between the outer fringe of the circle and the very heart of it. The plots signify some type of class, and when connecting them collectively, they’re like vertices that type shapes to assist see the connection of class values, not completely not like the vectors in an SVG.
Generally, the radar chart known as a spider chart, and it’s simple to see why. The axes that circulate outward intersect with the related plots and type a web-like look. So, in case your Spidey senses had been tingling at first look, you already know why.
You already know the place we’re going with this: We’re going to construct a radar chart collectively! We’ll work from scratch with nothing however HTML, CSS, and JavaScript. However earlier than we go there, it’s price noting a few issues about radar charts.
First, you don’t have to construct them from scratch. Chart.js and D3.js are available with handy approaches that vastly simplify the method. Seeing as I wanted only one chart for the mission, I made a decision towards utilizing a library and took on the problem of creating it myself. I discovered one thing new, and hopefully, you do as effectively!
Second, there are caveats to utilizing radar charts for knowledge visualization. Whereas they’re certainly efficient, they can be troublesome to learn when a number of collection stack up. The relationships between plots usually are not practically as decipherable as, say, bar charts. The order of the classes across the circle impacts the general form, and the dimensions between collection needs to be constant for drawing conclusions.
That each one stated, let’s dive in and get our fingers sticky with knowledge plots.
The Parts
The factor I like instantly about radar charts is that they’re inherently geometrical. Connecting plots produces a collection of angles that type polygon shapes. The edges are straight strains. And CSS is completely fantastic for working with polygons on condition that we’ve got the CSS polygon() operate for drawing them by declaring as many factors as we’d like within the operate’s arguments.
We are going to begin with a pentagonal-shaped chart with 5 knowledge classes.
See the Pen Radar chart (Pentagon) [forked] by Preethi Sam.
There are three elements we have to set up in HTML earlier than we work on styling. These could be:
Grids: These present the axes over which the diagrams are drawn. It’s the spider internet of the bunch.
Graphs: These are the polygons we draw with the coordinates of every knowledge plot earlier than coloring them in.
Labels: The textual content that identifies the classes alongside the graphs’ axes.
Right here’s how I made a decision to stub that out in HTML:
<!– GRIDS –>
<div class=”wrapper”>
<div class=”grids polygons”>
<div></div>
</div>
<div class=”grids polygons”>
<div></div>
</div>
<div class=”grids polygons”>
<div></div>
</div>
</div>
<!– GRAPHS –>
<div class=”wrapper”>
<div class=”graphs polygons”>
<div><!– Set 1 –></div>
</div>
<div class=”graphs polygons”>
<div><!– Set 2 –></div>
</div>
<div class=”graphs polygons”>
<div><!– Set 3 –></div>
</div>
<!– and so forth. –>
</div>
<!– LABELS –>
<div class=”wrapper”>
<div class=”labels”>Information A</div>
<div class=”labels”>Information B</div>
<div class=”labels”>Information C</div>
<div class=”labels”>Information D</div>
<div class=”labels”>Information E</div>
<!– and so forth. –>
</div>
I’m positive you may learn the markup and see what’s happening, however we’ve acquired three mother or father components (.wrapper) that every holds one of many most important elements. The primary mother or father incorporates the .grids, the second mother or father incorporates the .graphs, and the third mother or father incorporates the .labels.
Base Types
We’ll begin by organising just a few colour variables we are able to use to fill issues in as we go:
:root {
–color1: rgba(78, 36, 221, 0.6); /* graph set 1 */
–color2: rgba(236, 19, 154, 0.6); /* graph set 2 */
–color3: rgba(156, 4, 223, 0.6); /* graph set 3 */
–colorS: rgba(255, 0, 95, 0.1); /* graph shadow */
}
Our subsequent order of enterprise is to ascertain the format. CSS Grid is a stable method for this as a result of we are able to place all three grid gadgets collectively on the grid in simply a few strains:
/* Mother or father container */
.wrapper { show: grid; }
/* Inserting components on the grid */
.wrapper > div {
grid-area: 1 / 1; /* There’s just one grid space to cowl */
}
Let’s go forward and set a measurement on the grid gadgets. I’m utilizing a hard and fast size worth of 300px, however you should utilize any worth you want and variablize it in case you plan on utilizing it elsewhere. And fairly than declaring an specific peak, let’s put the burden of calculating a peak on CSS utilizing aspect-ratio to type good squares.
/* Inserting components on the grid */
.wrapper div {
aspect-ratio: 1 / 1;
grid-area: 1 / 1;
width: 300px;
}
We are able to’t see something simply but. We’ll want to paint issues in:
Graphs
———- */
.graphs:nth-of-type(1) > div { background: var(–color1); }
.graphs:nth-of-type(2) > div { background: var(–color2); }
.graphs:nth-of-type(3) > div { background: var(–color3); }
.graphs {
filter:
drop-shadow(1px 1px 10px var(–colorS))
drop-shadow(-1px -1px 10px var(–colorS))
drop-shadow(-1px 1px 10px var(–colorS))
drop-shadow(1px -1px 10px var(–colorS));
}
/* ————–
Grids
————– */
.grids {
filter:
drop-shadow(1px 1px 1px #ddd)
drop-shadow(-1px -1px 1px #ddd)
drop-shadow(-1px 1px 1px #ddd)
drop-shadow(1px -1px 1px #ddd);
mix-blend-mode: multiply;
}
.grids > div { background: white; }
Oh, wait! We have to set widths on the grids and polygons for them to take form:
.grids:nth-of-type(2) { width: 66%; }
.grids:nth-of-type(3) { width: 33%; }
/* ————–
Polygons
————– */
.polygons { place-self: heart; }
.polygons > div { width: 100%; }
Since we’re already right here, I’m going to place the labels a smidge and provides them width:
/* ————–
Labels
————– */
.labels:first-of-type { inset-block-sptart: -10%; }
.labels {
peak: 1lh;
place: relative;
width: max-content;
}
We nonetheless can’t see what’s happening, however we are able to if we quickly draw borders round components.
See the Pen Radar chart format [forked] by Preethi Sam.
All mixed, it doesn’t look all that nice to date. Mainly, we’ve got a collection of overlapping grids adopted by completely sq. graphs stacked proper on high of each other. The labels are off within the nook as effectively. We haven’t drawn something but, so this doesn’t hassle me for now as a result of we’ve got the HTML components we’d like, and CSS is technically establishing a format that ought to come collectively as we begin plotting factors and drawing polygons.
Extra particularly:
The .wrapper components are displayed as CSS Grid containers.
The direct kids of the .wrapper components are divs positioned in the very same grid-area. That is inflicting them to stack one proper on high of the opposite.
The .polygons are centered (place-self: heart).
The kid divs within the .polygons take up the total width (width:100%).
Each single div is 300px large and squared off with a one-to-one aspect-ratio.
We’re explicitly declaring a relative place on the .labels. This manner, they are often robotically positioned once we begin working in JavaScript.
The remainder? Merely apply some colours as backgrounds and drop shadows.
Calculating Plot Coordinates
Don’t fear. We’re not getting right into a deep dive about polygon geometry. As an alternative, let’s take a fast have a look at the equations we’re utilizing to calculate the coordinates of every polygon’s vertices. You don’t need to know these equations to make use of the code we’re going to jot down, nevertheless it by no means hurts to peek underneath the hood to see the way it comes collectively.
x1 = x + cosθ1 = cosθ1 if x=0
y1 = y + sinθ1 = sinθ1 if y=0
x2 = x + cosθ2 = cosθ2 if x=0
y2 = y + sinθ2 = sinθ2 if y=0
and so forth.
x, y = heart of the polygon (assigned (0, 0) in our examples)
x1, x2… = x coordinates of every vertex (vertex 1, 2, and so forth)
y1, y2… = y coordinates of every vertex
θ1, θ2… = angle every vertex makes to the x-axis
We are able to assume that 𝜃 is 90deg (i.e., 𝜋/2) since a vertex can at all times be positioned proper above or beneath the middle (i.e., Information A on this instance). The remainder of the angles might be calculated like this:
n = variety of sides of the polygon
𝜃1 = 𝜃0 + 2𝜋/𝑛 = 𝜋/2 + 2𝜋/𝑛
𝜃2 = 𝜃0 + 4𝜋/𝑛 = 𝜋/2 + 4𝜋/𝑛
𝜃3 = 𝜃0 + 6𝜋/𝑛 = 𝜋/2 + 6𝜋/𝑛
𝜃3 = 𝜃0 + 8𝜋/𝑛 = 𝜋/2 + 8𝜋/𝑛
𝜃3 = 𝜃0 + 10𝜋/𝑛 = 𝜋/2 + 10𝜋/𝑛
Armed with this context, we are able to remedy for our x and y values:
x1 = cos(𝜋/2 + 2𝜋/# sides)
y1 = sin(𝜋/2 + 2𝜋/# sides)
x2 = cos(𝜋/2 + 4𝜋/# sides)
y2 = sin(𝜋/2 + 4𝜋/# sides)
and so forth.
The variety of sides will depend on the variety of plots we’d like. We stated up-front that it is a pentagonal form, so we’re working with 5 sides on this specific instance.
x1 = cos(𝜋/2 + 2𝜋/5)
y1 = sin(𝜋/2 + 2𝜋/5)
x2 = cos(𝜋/2 + 4𝜋/5)
y2 = sin(𝜋/2 + 4𝜋/5)
and so forth.
Drawing Polygons With JavaScript
Now that the maths is accounted for, we’ve got what we have to begin working in JavaScript for the sake of plotting the coordinates, connecting them collectively, and portray within the ensuing polygons.
For simplicity’s sake, we are going to go away the Canvas API out of this and as a substitute use common HTML components to attract the chart. You’ll be able to, nevertheless, use the maths outlined above and the next logic as the muse for drawing polygons in whichever language, framework, or API you like.
OK, so we’ve got three varieties of elements to work on: grids, graphs, and labels. We begin with the grid and work up from there. In every case, I’ll merely drop within the code and clarify what’s occurring.
Drawing The Grid
let sides = 5; // # of information factors
let models = 1; // # of graphs + 1
let vertices = (new Array(models)).fill(“”);
let percents = new Array(models);
percents[0] = (new Array(sides)).fill(100); // for the polygon’s grid element
let gradient = “conic-gradient(“;
let angle = 360/sides;
// Calculate vertices
with(Math) {
for(i=0, n = 2 * PI; i < sides; i++, n += 2 * PI) {
for(j=0; j < models; j++) {
let x = ( spherical(cos(-1 * PI/2 + n/sides) * percents[j][i]) + 100 ) / 2;
let y = ( spherical(sin(-1 * PI/2 + n/sides) * percents[j][i]) + 100 ) / 2;
vertices[j] += ${x}% ${y} ${i == sides – 1 ? ‘%’:’%, ‘};
}
gradient += white ${
(angle * (i+1)) – 1}deg,
#ddd ${ (angle * (i+1)) – 1 }deg,
#ddd ${ (angle * (i+1)) + 1 }deg,
white ${ (angle * (i+1)) + 1 }deg,;}
}
// Draw the grids
doc.querySelectorAll(‘.grids>div’).forEach((grid,i) => {
grid.fashion.clipPath =polygon(${ vertices[0] });
});
doc.querySelector(‘.grids:nth-of-type(1) > div’).fashion.background =${gradient.slice(0, -1)} );
Test it out! We have already got a spider internet.
See the Pen Radar chart (Grid) [forked] by Preethi Sam.
Right here’s what’s occurring within the code:
sides is the variety of sides of the chart. Once more, we’re working with 5 sides.
vertices is an array that shops the coordinates of every vertex.
Since we’re not establishing any graphs but — solely the grid — the variety of models is about to 1, and just one merchandise is added to the percents array at percents[0]. For grid polygons, the info values are 100.
gradient is a string to assemble the conic-gradient() that establishes the grid strains.
angle is a calculation of 360deg divided by the full variety of sides.
From there, we calculate the vertices:
i is an iterator that cycles by the full variety of sides (i.e., 5).
j is an iterator that cycles by the full variety of models (i.e., 1).
n is a counter that counts in increments of two*PI (i.e., 2𝜋, 4𝜋, 6𝜋, and so forth).
The x and y values of every vertex are calculated as follows, primarily based on the geometric equations we mentioned earlier. Observe that we multiply 𝜋 by -1 to steer the rotation.
sin(-1 * PI/2 + n/sides) // sin(𝜋/2 + 2𝜋/sides), sin(𝜋/2 + 4𝜋/sides)…
We convert the x and y values into percentages (since that’s how the info factors are formatted) after which place them on the chart.
let y = (spherical(sin(-1 * PI/2 + n/sides) * percents[j][i]) + 100) / 2;
We additionally assemble the conic-gradient(), which is a part of the grid. Every colour cease corresponds to every vertex’s angle — at every of the angle increments, a gray (#ddd) line is drawn.
gradient +=
`white ${ (angle * (i+1)) – 1 }deg,
#ddd ${ (angle * (i+1)) – 1 }deg,
#ddd ${ (angle * (i+1)) + 1 }deg,
white ${ (angle * (i+1)) + 1 }deg,`
If we print out the computed variables after the for loop, these would be the outcomes for the grid’s vertices and gradient:
// polygon(97.5% 34.5%, 79.5% 90.5%, 20.5% 90.5%, 2.5% 34.5%, 50% 0%)
console.log(gradient.slice(0, -1)); /* grid’s gradient */
// conic-gradient(white 71deg, #ddd 71deg,# ddd 73deg, white 73deg, white 143deg, #ddd 143deg, #ddd 145deg, white 145deg, white 215deg, #ddd 215deg, #ddd 217deg, white 217deg, white 287deg, #ddd 287deg, #ddd 289deg, white 289deg, white 359deg, #ddd 359deg, #ddd 361deg, white 361deg
These values are assigned to the grid’s clipPath and background, respectively, and thus the grid seems on the web page.
The Graph
// Every graph’s knowledge factors within the order [B, C, D… A] percents[1] = [100, 50, 60, 50, 90];
percents[2] = [100, 80, 30, 90, 40];
percents[3] = [100, 10, 60, 60, 80];
// Subsequent to drawing grids
doc.querySelectorAll(‘.graphs > div’).forEach((graph,i) => {
graph.fashion.clipPath =polygon( ${vertices[i+1]} );
});
See the Pen Radar chart (Graph) [forked] by Preethi Sam.
Now it appears like we’re getting someplace! For every graph, we add its set of information factors to the percents array after incrementing the worth of models to match the variety of graphs. And that’s all we have to draw graphs on the chart. Let’s flip our consideration to the labels for the second.
The Labels
// First label is at all times set within the high center
let firstLabel = doc.querySelector(‘.labels:first-of-type’);
firstLabel.fashion.insetInlineStart =calc(50% – ${firstLabel.offsetWidth / 2}px);
// Setting labels for the remainder of the vertices (knowledge factors).
let v = Array.from(vertices[0].break up(‘ ‘).splice(0, (2 * sides) – 2), (n)=> parseInt(n));
doc.querySelectorAll(‘.labels:not(:first-of-type)’).forEach((label, i) => {
let width = label.offsetWidth / 2;
let peak = label.offsetHeight;
label.fashion.insetInlineStart = calc( ${ v[i*2] }% + ${ v[i*2] < 50 ? – 3*width : v[i*2] == 50 ? – width: width}px );
label.fashion.insetBlockStart = calc( ${ v[(i*2) + 1] }% – ${ v[(i * 2) + 1] == 100 ? – peak: peak / 2 }px );
});
The positioning of the labels is decided by three issues:
The coordinates of the vertices (i.e., knowledge factors) they need to be subsequent to,
The width and peak of their textual content, and
Any clean house wanted across the labels so that they don’t overlap the chart.
All of the labels are positioned relative in CSS. By including the inset-inline-start and inset-block-start values within the script, we are able to reposition the labels utilizing the values as coordinates. The primary label is at all times set to the top-middle place. The coordinates for the remainder of the labels are the identical as their respective vertices, plus an offset. The offset is decided like this:
x-axis/horizontal
If the label is on the left (i.e., x is lower than 50%), then it’s moved in direction of the left primarily based on its width. In any other case, it’s moved in direction of the fitting facet. As such, the fitting or left edges of the labels, relying on which facet of the chart they’re on, are uniformly aligned to their vertices.
y-axis/vertical
The peak of every label is mounted. There’s not a lot offset so as to add besides perhaps shifting them down half their peak. Any label on the backside (i.e., when y is 100%), nevertheless, might use further house above it for respiration room.
And guess what…
We’re Accomplished!
See the Pen Radar chart (Pentagon) [forked] by Preethi Sam.
Not too shabby, proper? Probably the most sophisticated half, I believe, is the maths. However since we’ve got that found out, we are able to virtually plug it into every other state of affairs the place a radar chart is required. Want a four-point chart as a substitute? Replace the variety of vertices within the script and account for fewer components within the markup and kinds.
In actual fact, listed here are two extra examples displaying completely different configurations. In every case, I’m merely growing or reducing the variety of vertices, which the script makes use of to supply completely different units of coordinates that assist place factors alongside the grid.
Want simply three sides? All which means is 2 fewer coordinate units:
See the Pen Radar chart (Triangle) [forked] by Preethi Sam.
Want seven sides? We’ll produce extra coordinate units as a substitute:
See the Pen Radar chart (Heptagon) [forked] by Preethi Sam.
Subscribe to MarketingSolution.
Receive web development discounts & web design tutorials.
Now! Lets GROW Together!