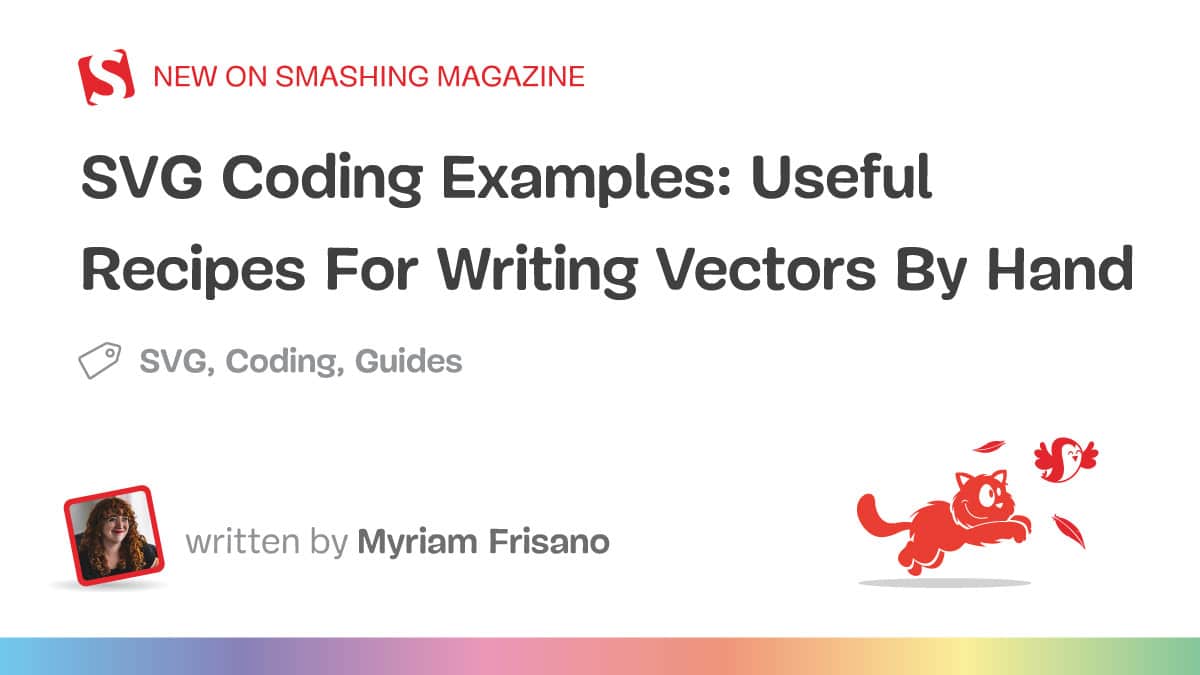
Though I’m the type of front-end engineer who manually cleans up SVG recordsdata when they’re a multitude, I by no means anticipated to turn out to be certainly one of these individuals. You realize, these loopy folks that draw with code.
However right here we’re.
I dove deep into SVG specs final winter after I created a challenge to draw Calligraphy Grids, and regardless that I knew the essential buildings and guidelines of SVG, it was solely then that I totally tried to determine and perceive what all of these numbers meant and the way they interacted with one another.
And, when you get the cling of it, it’s truly very attention-grabbing and fairly enjoyable to code SVG by hand.
No <path> forward
We received’t go into extra advanced SVG shapes like paths on this article, that is extra about sensible data for easy SVGs. Relating to drawing curves, I nonetheless advocate utilizing a software like Illustrator or Affinity. Nonetheless, if you’re tremendous into compounding your traces, a path is beneficial. Possibly we’ll do this in Half 2.
Additionally, this information focuses totally on sensible examples that illustrate a number of the math concerned when drawing SVGs. There’s a great article right here that goes a bit deeper into the specs, which I like to recommend studying in the event you’re extra concerned with that: “A Sensible Information To SVG And Design Instruments.”
Drawing With Math. Bear in mind Coordinate Techniques?
Illustrator, Affinity, and all different vector applications are mainly simply serving to you draw on a coordinate system, after which these paths and shapes are saved in SVG recordsdata.
Should you open up these recordsdata in an editor, you’ll see that they’re only a bunch of paths that comprise a number of numbers, that are coordinates in that coordinate system that make up the traces.
However, there’s a distinction between the omnipotent <path> and the opposite, extra semantic components like <rect>, <circle>, <line>, <ellipse>, <polygon>, and <polyline>.
These components will not be that arduous to learn and write by hand, they usually open up a number of prospects so as to add animation and different enjoyable stuff. So, whereas most individuals would possibly solely consider SVGs as never-pixelated, infinitely scaling photographs, they will also be fairly complete items of code.
How Does SVG Work? unit != unit
Earlier than we get began on how SVG components are drawn, let’s discuss concerning the methods items work in SVG as a result of they is perhaps a bit complicated whenever you first get began.
The great thing about SVG is that it’s a vector format, which signifies that the items are considerably indifferent from the browser and are as an alternative simply relative to the coordinate system you’re working in.
Which means you’ll not use a unit inside SVG however quite simply use numbers after which outline the scale of the doc you’re working with.
So, your width and peak is perhaps utilizing CSS rem items, however in your viewBox, items turn out to be only a idea that helps you in establishing sizing relationships.
What Is The viewBox?
The viewBox works a little bit bit just like the CSS aspect-ratio property. It helps you determine a relationship between the width and the peak of your coordinate system and units up the field you’re working in. I have a tendency to think about the viewBox as my “doc” measurement.
Any aspect that’s positioned throughout the SVG with larger dimensions than the viewBox is not going to be seen. So, the viewBox is the cutout of the coordinate system we’re wanting by way of. The width and peak attributes are pointless if there’s a viewBox attribute.
So, in brief, having an SVG with a viewBox makes it behave so much like a daily picture. And identical to with photographs, it’s often best to only set both a width or a peak and let the opposite dimension be routinely sized based mostly on the intrinsic facet ratio dimensions.
So, if we have been to create a perform that pulls an SVG, we’d retailer three separate variables and fill them in like this:
`<svg
width=”${svgWidth}”
viewBox=”0 0 ${documentWidth} ${documentHeight}”
xmlns=”http://www.w3.org/2000/svg”
>`;
SVG Issues Of Be aware
There’s a lot to learn about SVG: If you wish to reuse a picture so much, it’s possible you’ll wish to flip it into a logo that may then be referenced with a use tag, you may create sprites, and there are some greatest practices when utilizing them for icons, and so forth.
Sadly, this can be a bit out of the scope of this text. Right here, we’re primarily specializing in designing SVG recordsdata and never on how we will optimize and use them.
Nonetheless, one factor of be aware that’s simpler to implement from the beginning is accessibility.
SVGs can be utilized in an <img> tag, the place alt tags can be found, however then you definitely lose the power to work together along with your SVG code, so inlining is perhaps your choice.
When inlining, it’s best to declare position=”img” after which add a <title> tag along with your picture title.
Be aware: You may take a look at this text for SVG and Accessibility suggestions.
<svg
position=”img”
[…attr]
>
<title>An accessible title</title>
<!– design code –>
</svg>
Drawing SVG With JavaScript
There may be often some arithmetic concerned when drawing SVGs. It’s often pretty easy arithmetic (besides, you already know, in case you draw calligraphy grids after which need to dig out trigonometry…), however I believe even for easy math, most individuals don’t write their SVGs in pure HTML and thus wish to use algebra.
A minimum of for me, I discover it a lot simpler to know SVG Code when giving which means to numbers, so I all the time persist with JavaScript, and by giving my coordinates names, I like them immeasurable instances extra.
So, for the upcoming examples, we’ll take a look at the listing of variables with the straightforward math after which JSX-style templates for interpolation, as that offers extra legible syntax highlighting than string interpolations, after which every instance might be obtainable as a CodePen.
To maintain this Information framework-agnostic, I wished to shortly go over drawing SVG components with simply good outdated vanilla JavaScript.
We’ll create a container aspect in HTML that we will put our SVG into and seize that aspect with JavaScript.
<div data-svg-container></div>
<script src=”template.js”></script>
To make it easy, we’ll draw a rectangle <rect> that covers your complete viewBox and makes use of a fill.
Be aware: You may add all legitimate CSS values as fills, so a set coloration, or one thing like currentColor to entry the positioning’s textual content coloration or a CSS variable would work right here in the event you’re inlining your SVG and wish it to work together with the web page it’s positioned in.
Let’s first begin with our variable setup.
const container = doc.querySelector(“[data-svg-container]”);
const svgWidth = “30rem”; // use any worth with items right here
const documentWidth = 100;
const documentHeight = 100;
const rectWidth = documentWidth;
const rectHeight = documentHeight;
const rectFill = “currentColor”; // use any coloration worth right here
const title = “A easy sq. field”;
Methodology 1: Create Aspect and Set Attributes
This methodology is less complicated to maintain type-safe (if utilizing TypeScript) — makes use of correct SVG components and attributes, and so forth — however it’s much less performant and will take a very long time when you’ve got many components.
const titleElement = doc.createElementNS(“http://www.w3.org/2000/svg”, “title”);
const rect = doc.createElementNS(“http://www.w3.org/2000/svg”, “rect”);
svg.setAttribute(“width”, svgWidth);
svg.setAttribute(“viewBox”, 0 0 ${documentWidth} ${documentHeight});
svg.setAttribute(“xmlns”, “http://www.w3.org/2000/svg”);
svg.setAttribute(“position”, “img”);
titleElement.textContent = title;
rect.setAttribute(“width”, rectWidth);
rect.setAttribute(“peak”, rectHeight);
rect.setAttribute(“fill”, rectFill);
svg.appendChild(titleElement);
svg.appendChild(rect);
container.appendChild(svg);
Right here, you may see that with the identical coordinates, a polyline received’t draw the road between the blue and the purple dot, whereas a polygon will. Nonetheless, when making use of a fill, they take the very same data as if the form was closed, which is the appropriate facet of the graphic, the place the polyline makes it seem like a bit of a circle is lacking.
That is the second time the place now we have handled fairly a little bit of repetition, and we will take a look at how we might leverage the ability of JavaScript logic to render our template sooner.
However first, we’d like a fundamental implementation like we’ve carried out earlier than. We’re creating objects for the circles, after which we’re chaining the cx and cy values collectively to create the factors attribute. We’re additionally storing our transforms in variables.
const polyDocHeight = 200;
const circleOne = { cx: 25, cy: 80, r: 10, fill: “purple” };
const circleTwo = { cx: 40, cy: 20, r: 5, fill: “lime” };
const circleThree = { cx: 70, cy: 60, r: 8, fill: “cyan” };
const factors = ${circleOne.cx},${circleOne.cy} ${circleTwo.cx},${circleTwo.cy} ${circleThree.cx},${circleThree.cy};
const moveToTopRight = translate(${polyDocWidth / 2}, 0);
const moveToBottomRight = translate(${polyDocWidth / 2}, ${polyDocHeight / 2});
const moveToBottomLeft = translate(0, ${polyDocHeight / 2});
After which, we apply the variables to the template, utilizing both a polyline or polygon aspect and a fill attribute that’s both set to none or a coloration worth.
<svg
width={svgWidth}
viewBox={`0 0 ${polyDocWidth} ${polyDocHeight}`}
xmlns=”http://www.w3.org/2000/svg”
position=”img”
>
<title>Composite form comparability</title>
<g>
<circle
cx={circleOne.cx}
cy={circleOne.cy}
r={circleOne.r}
fill={circleOne.fill}
/>
<circle
cx={circleTwo.cx}
cy={circleTwo.cy}
r={circleTwo.r}
fill={circleTwo.fill}
/>
<circle
cx={circleThree.cx}
cy={circleThree.cy}
r={circleThree.r}
fill={circleThree.fill}
/>
<polyline
factors={factors}
fill=”none”
stroke=”black”
/>
</g>
<g remodel={moveToTopRight}>
<circle
cx={circleOne.cx}
cy={circleOne.cy}
r={circleOne.r}
fill={circleOne.fill}
/>
<circle
cx={circleTwo.cx}
cy={circleTwo.cy}
r={circleTwo.r}
fill={circleTwo.fill}
/>
<circle
cx={circleThree.cx}
cy={circleThree.cy}
r={circleThree.r}
fill={circleThree.fill}
/>
<polyline
factors={factors}
fill=”white”
stroke=”black”
/>
</g>
<g remodel={moveToBottomLeft}>
<circle
cx={circleOne.cx}
cy={circleOne.cy}
r={circleOne.r}
fill={circleOne.fill}
/>
<circle
cx={circleTwo.cx}
cy={circleTwo.cy}
r={circleTwo.r}
fill={circleTwo.fill}
/>
<circle
cx={circleThree.cx}
cy={circleThree.cy}
r={circleThree.r}
fill={circleThree.fill}
/>
<polygon
factors={factors}
fill=”none”
stroke=”black”
/>
</g>
<g remodel={moveToBottomRight}>
<circle
cx={circleOne.cx}
cy={circleOne.cy}
r={circleOne.r}
fill={circleOne.fill}
/>
<circle
cx={circleTwo.cx}
cy={circleTwo.cy}
r={circleTwo.r}
fill={circleTwo.fill}
/>
<circle
cx={circleThree.cx}
cy={circleThree.cy}
r={circleThree.r}
fill={circleThree.fill}
/>
<polygon
factors={factors}
fill=”white”
stroke=”black”
/>
</g>
</svg>
And right here’s a model of it to play with:
See the Pen SVG Polygon / Polyline (easy) [forked] by Myriam.
Dealing With Repetition
Relating to drawing SVGs, it’s possible you’ll discover that you simply’ll be repeating a number of the identical code over and over. That is the place JavaScript can come in useful, so let’s take a look at the composite instance once more and see how we might optimize it so that there’s much less repetition.
Observations:
Now we have three circle components, all following the identical sample.
We create one repetition to alter the fill model for the aspect.
We repeat these two components yet one more time, with both a polyline or a polygon.
Now we have 4 completely different transforms (technically, no remodel is a remodel on this case).
This tells us that we will create nested loops.
Let’s return to only a vanilla implementation for this because the method loops are carried out is kind of completely different throughout frameworks.
You would make this extra generic and write separate generator features for every kind of aspect, however that is simply to offer you an thought of what you can do by way of logic. There are definitely nonetheless methods to optimize this.
I’ve opted to have arrays for every kind of variation that now we have and wrote a helper perform that goes by way of the info and builds out an array of objects with all the required data for every group. In such a brief array, it will definitely be a viable choice to only have the info saved in a single aspect, the place the values are repeated, however we’re taking the DRY factor critically on this one.
The group array can then be looped over to construct our SVG HTML.
const svgWidth = 200;
const documentWidth = 200;
const documentHeight = 200;
const halfWidth = documentWidth / 2;
const halfHeight = documentHeight / 2;
const circles = [
{ cx: 25, cy: 80, r: 10, fill: “red” },
{ cx: 40, cy: 20, r: 5, fill: “lime” },
{ cx: 70, cy: 60, r: 8, fill: “cyan” },
];
const factors = circles.map(({ cx, cy }) => ${cx},${cy}).be a part of(” “);
const components = [“polyline”, “polygon”];
const fillOptions = [“none”, “white”];
const transforms = [
undefined,
translate(${halfWidth}, 0),
translate(0, ${halfHeight}),
translate(${halfWidth}, ${halfHeight}),
];
const makeGroupsDataObject = () => {
let counter = 0;
const g = [];
components.forEach((aspect) => {
fillOptions.forEach((fill) => {
const remodel = transforms[counter++];
g.push({ aspect, fill, remodel });
});
});
return g;
};
const teams = makeGroupsDataObject();
// outcome:
// [
// {
// element: “polyline”,
// fill: “none”,
// },
// {
// element: “polyline”,
// fill: “white”,
// transform: “translate(100, 0)”,
// },
// {
// element: “polygon”,
// fill: “none”,
// transform: “translate(0, 100)”,
// },
// {
// element: “polygon”,
// fill: “white”,
// transform: “translate(100, 100)”,
// }
// ]
const svg = doc.createElementNS(“http://www.w3.org/2000/svg”, “svg”);
svg.setAttribute(“width”, svgWidth);
svg.setAttribute(“viewBox”, 0 0 ${documentWidth} ${documentHeight});
svg.setAttribute(“xmlns”, “http://www.w3.org/2000/svg”);
svg.setAttribute(“position”, “img”);
svg.innerHTML = “<title>Composite form comparability</title>”;
teams.forEach((groupData) => {
const circlesHTML = circles
.map((circle) => {
return <circle
cx=”${circle.cx}”
cy=”${circle.cy}”
r=”${circle.r}”
fill=”${circle.fill}”
/>;
})
.be a part of(“”);
const polyElementHTML = <${groupData.aspect}
factors=”${factors}”
fill=”${groupData.fill}”
stroke=”black”
/>;
const group = <g ${groupData.remodel ?remodel=”${groupData.remodel}”: “”}>
${circlesHTML}
${polyElementHTML}
</g>;
svg.innerHTML += group;
});
container.appendChild(svg);
And right here’s the Codepen of that:
See the Pen SVG Polygon / Polyline (JS loop model) [forked] by Myriam.
Extra Enjoyable Stuff
Now, that’s all of the fundamentals I wished to cowl, however there may be a lot extra you are able to do with SVG. There may be extra you are able to do with remodel; you should use a masks, you should use a marker, and so forth.
We don’t have time to dive into all of them at this time, however since this began for me when making Calligraphy Grids, I wished to indicate you the 2 most satisfying ones, which I, sadly, can’t use within the generator since I wished to have the ability to open my generated SVGs in Affinity and it doesn’t assist sample.
Okay, so sample is a part of the defs part throughout the SVG, which is the place you may outline reusable components that you would be able to then reference in your SVG.
Graph Grid with sample
If you concentrate on it, a graph is only a bunch of horizontal and vertical traces that repeat throughout the x- and y-axis.
So, sample will help us with that. We are able to create a <rect> after which reference a sample within the fill attribute of the rect. The sample then has its personal width, peak, and viewBox, which defines how the sample is repeated.
So, let’s say we wish to completely heart our graph grid in any given width or peak, and we wish to have the ability to outline the scale of our ensuing squares (cells).
As soon as once more, let’s begin with the JavaScipt variables:
const graphDocWidth = 226;
const graphDocHeight = 101;
const cellSize = 5;
const strokeWidth = 0.3;
const strokeColor = “currentColor”;
const patternHeight = (cellSize / graphDocHeight) * 100;
const patternWidth = (cellSize / graphDocWidth) * 100;
const gridYStart = (graphDocHeight % cellSize) / 2;
const gridXStart = (graphDocWidth % cellSize) / 2;
Now, we will apply them to the SVG aspect:
<svg
width={svgWidth}
viewBox={`0 0 ${graphDocWidth} ${graphDocHeight}`}
xmlns=”http://www.w3.org/2000/svg”
position=”img”
>
<defs>
<sample
id=”horizontal”
viewBox={`0 0 ${graphDocWidth} ${strokeWidth}`}
width=”100%”
peak={`${patternHeight}%`}
>
<line
x1=”0″
x2={graphDocWidth}
y1={gridYStart}
y2={gridYStart}
stroke={strokeColor}
stroke-width={strokeWidth}
/>
</sample>
<sample
id=”vertical”
viewBox={`0 0 ${strokeWidth} ${graphDocHeight}`}
width={`${patternWidth}%`}
peak=”100%”
>
<line
y1={0}
y2={graphDocHeight}
x1={gridXStart}
x2={gridXStart}
stroke={strokeColor}
stroke-width={strokeWidth}
/>
</sample>
</defs>
<title>A graph grid</title>
<rect
width={graphDocWidth}
peak={graphDocHeight}
fill=”url(#horizontal)”
/>
<rect
width={graphDocWidth}
peak={graphDocHeight}
fill=”url(#vertical)”
/>
</svg>
And that is what that then seems to be like:
See the Pen SVG Graph Grid [forked] by Myriam.
Dot Grid With sample
If we wished to attract a dot grid as an alternative, we might merely repeat a circle. Or, we might alternatively use a line with a stroke-dasharray and stroke-dashoffset to create a dashed line. And we’d solely want one line on this case.
Beginning with our JavaScript variables:
const dotDocWidth = 219;
const dotDocHeight = 100;
const cellSize = 4;
const strokeColor = “black”;
const gridYStart = (dotDocHeight % cellSize) / 2;
const gridXStart = (dotDocWidth % cellSize) / 2;
const dotSize = 0.5;
const patternHeight = (cellSize / dotDocHeight) * 100;
After which including them to the SVG aspect:
<svg
width={svgWidth}
viewBox={`0 0 ${dotDocWidth} ${dotDocHeight}`}
xmlns=”http://www.w3.org/2000/svg”
position=”img”
>
<defs>
<sample
id=”horizontal-dotted-line”
viewBox={`0 0 ${dotDocWidth} ${dotSize}`}
width=”100%”
peak={`${patternHeight}%`}
>
<line
x1={gridXStart}
y1={gridYStart}
x2={dotDocWidth}
y2={gridYStart}
stroke={strokeColor}
stroke-width={dotSize}
stroke-dasharray={`0,${cellSize}`}
stroke-linecap=”spherical”
></line>
</sample>
</defs>
<title>A Dot Grid</title>
<rect
x=”0″
y=”0″
width={dotDocWidth}
peak={dotDocHeight}
fill=”url(#horizontal-dotted-line)”
></rect>
</svg>
And that is what that appears like:
See the Pen SVG Dot Grid [forked] by Myriam.
Conclusion
This brings us to the top of our little introductory journey into SVG. As you may see, coding SVG by hand is just not as scary because it appears. Should you break it down into the essential components, it turns into fairly like some other coding job:
We analyze the issue,
Break it down into smaller components,
Study every coordinate and its mathematical breakdown,
After which put all of it collectively.
I hope that this text has given you a place to begin into the great world of coded photographs and that it offers you the motivation to delve deeper into the specs and check out drawing some your self.
Subscribe to MarketingSolution.
Receive web development discounts & web design tutorials.
Now! Lets GROW Together!